# Titanium.UI.iOS.DynamicItemBehavior
Base dynamic configuration for an item.
# Overview
A dynamic item behavior configures the physics attributes for one or more items. These attributes, such as density and resistance, affects the behavior of the object when other behaviors, such as push forces or collisions, are applied to it. To define a dynamic behavior for an item:
- Use the Titanium.UI.iOS.createDynamicItemBehavior method to create and define the behavior.
- Use the Titanium.UI.iOS.DynamicItemBehavior.addItem method to add items to the behavior.
- Add the behavior to an Titanium.UI.iOS.Animator.
# Examples
# Simple Example
The following example create two blocks, which are pushed towards each other. Because the red block is more dense and has higher resistance than the blue block, the red block moves steadily to the left, while the blue block spins around unpredictably.
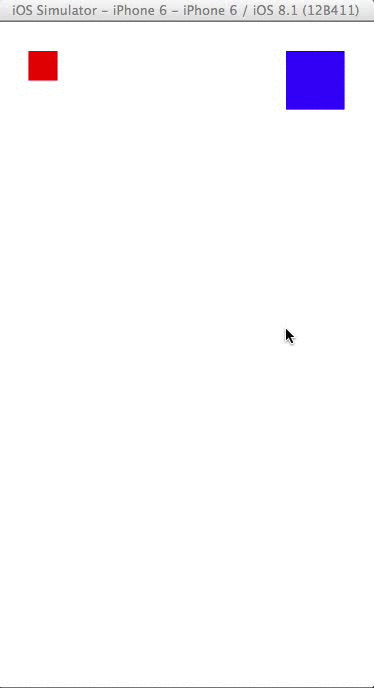
var win = Ti.UI.createWindow({backgroundColor: 'white', fullscreen: true});
// Create an Animator object using the window as the coordinate system
var animator = Ti.UI.iOS.createAnimator({referenceView: win});
// Create a red block
var redBlock = Ti.UI.createView({
backgroundColor: 'red',
width: 25,
height: 25,
top: 25,
left: 25
});
// Change the physics attributes of the red block
var redDynamic = Ti.UI.iOS.createDynamicItemBehavior({
density: 20.0,
angularResistance: 1.0,
friction: 1.0,
resistance: 1.0,
allowsRotation: false
});
redDynamic.addItem(redBlock);
// Apply a left push to the red block
var redPush = Ti.UI.iOS.createPushBehavior({
pushDirection: {x: 2.0, y: 0.0}
});
redPush.addItem(redBlock);
// Create a blue block
var blueBlock = Ti.UI.createView({
backgroundColor: 'blue',
width: 50,
height: 50,
top: 25,
right: 25
});
// Change the physics attributes of the blue block
var blueDynamic = Ti.UI.iOS.createDynamicItemBehavior({
elasticity: 1.0,
});
blueDynamic.addItem(blueBlock);
// Apply a right push to the blue block
var bluePush = Ti.UI.iOS.createPushBehavior({
pushDirection: {x: -2.0, y: 0.0}
});
bluePush.addItem(blueBlock);
// Create the collision behavior so the items can collide
var collision = Ti.UI.iOS.createCollisionBehavior();
collision.addItem(redBlock);
collision.addItem(blueBlock);
animator.addBehavior(redDynamic);
animator.addBehavior(redPush);
animator.addBehavior(blueDynamic);
animator.addBehavior(bluePush);
animator.addBehavior(collision);
// Start the animation when the window opens
win.addEventListener('open', function(e){
animator.startAnimator();
});
win.add(redBlock);
win.add(blueBlock);
win.open();
# Properties
# allowsRotation
Specifies if this item can rotate.
Set to true
to enable this behavior or false
to disable.
Default: true
# angularResistance
Specifies the angular resistance of this item.
The greater the value, the greater the angular damping and rotation slows to a stop faster.
Default: 0
# apiName READONLY
The name of the API that this proxy corresponds to.
The value of this property is the fully qualified name of the API. For example, Titanium.UI.Button
returns Ti.UI.Button
.
# bubbleParent
Indicates if the proxy will bubble an event to its parent.
Some proxies (most commonly views) have a relationship to other proxies, often established by the add() method. For example, for a button added to a window, a click event on the button would bubble up to the window. Other common parents are table sections to their rows, table views to their sections, and scrollable views to their views. Set this property to false to disable the bubbling to the proxy's parent.
Default: true
# density
Specifies the relative mass density of this item.
An item's density along with its size determines its effective mass and affects its dynamic behavior.
Default: 1
# elasticity
Specifies the elasticity applied to collisions for this item.
A value of 0.0 indicates no bounce upon collision, while 1.0 indicates a completely elastic collision.
Default: 0
# friction
Specifies the linear resistance of the item when it slides against another item.
A value of 0.0 indicates no friction, while 1.0 indicates strong friction. Use higher numbers to apply even higher friction.
Default: 0
# resistance
Specifies the linear resistance of this item which reduces linear velocity over time.
A value of 0.0 indicates no velocity damping.
Default: 0
# Methods
# addAngularVelocityForItem
Adds a specified angular velocity for the item.
Parameters
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | Item to add the velocity for. |
velocity | Number | Velocity to add or subtract in radians per second. If the current velocity is positive, the item spins clockwise. A negative value means the item spins counter-clockwise. |
Returns
- Type
- void
# addEventListener
Adds the specified callback as an event listener for the named event.
Parameters
Name | Type | Description |
---|---|---|
name | String | Name of the event. |
callback | Callback<Titanium.Event> | Callback function to invoke when the event is fired. |
Returns
- Type
- void
# addItem
Adds an item to this behavior.
Parameters
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | View object to add to the behavior. |
Returns
- Type
- void
# addLinearVelocityForItem
Adds a specified linear velocity for the item.
Parameters
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | Item to add the velocity for. |
velocity | Point | Velocity to add or substract in points per second in the x and y directions. |
Returns
- Type
- void
# angularVelocityForItem
Returns the angular velocity of the item.
Parameters
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | Item to retrieve the velocity of. |
Returns
- Type
- Number
# applyProperties
Applies the properties to the proxy.
Properties are supplied as a dictionary. Each key-value pair in the object is applied to the proxy such that myproxy[key] = value.
Parameters
Name | Type | Description |
---|---|---|
props | Dictionary | A dictionary of properties to apply. |
Returns
- Type
- void
# fireEvent
Fires a synthesized event to any registered listeners.
Parameters
Name | Type | Description |
---|---|---|
name | String | Name of the event. |
event | Dictionary | A dictionary of keys and values to add to the Titanium.Event object sent to the listeners. |
Returns
- Type
- void
# linearVelocityForItem
Returns the linear velocity of the item.
Parameters
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | Item to retrieve the velocity of. |
Returns
- Type
- Point
# removeEventListener
Removes the specified callback as an event listener for the named event.
Multiple listeners can be registered for the same event, so the
callback
parameter is used to determine which listener to remove.
When adding a listener, you must save a reference to the callback function in order to remove the listener later:
var listener = function() { Ti.API.info("Event listener called."); }
window.addEventListener('click', listener);
To remove the listener, pass in a reference to the callback function:
window.removeEventListener('click', listener);
Parameters
Name | Type | Description |
---|---|---|
name | String | Name of the event. |
callback | Callback<Titanium.Event> | Callback function to remove. Must be the same function passed to |
Returns
- Type
- void
# removeItem
Removes the specified item from this behavior.
Parameters
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | Item to remove. |
Returns
- Type
- void